Custom Sign-in Page
To add a custom sign-in page, you’ll need to define the path to your page in the pages
object in your Auth.js configuration. Make sure a route / page actually exists at the path you’re defining here!
./auth.ts
import NextAuth from "next-auth"
import GitHub from "next-auth/providers/github"
import type { NextAuthConfig } from "next-auth"
// Define your configuration in a separate variable and pass it to NextAuth()
// This way we can also 'export const config' for use later
export const config = {
providers: [GitHub],
pages: {
signIn: "/signin",
},
} satisfies NextAuthConfig
export const { handlers, auth, signIn, signOut } = NextAuth(config)
We can now build our own custom sign in page:
app/signin/page.tsx
import { signIn, config, auth } from "../../../auth.ts"
import { redirect } from "next/navigation"
async function getSession() {
const session = await auth()
return {
session,
}
}
export default async function SignInPage() {
const { session } = await getSession()
// The user is already logged in, redirect to homepage.
// Make sure is not the same URL to avoid an infinite loop!
if (session) return redirect("/")
return (
<div className="flex flex-col gap-4 max-w-80 mx-auto justify-center h-screen">
{Object.values(config.providers).map((provider) => (
<form action={provider.authorization.url} method="POST">
<Button className="flex flex-row gap-2" type="submit">
<span>Sign in with {provider.name}</span>
</Button>
</form>
))}
</div>
)
}
Then when clicking on “Sign in” on the app (calling signIn
), our custom sign in page will appear with the available sign in options:
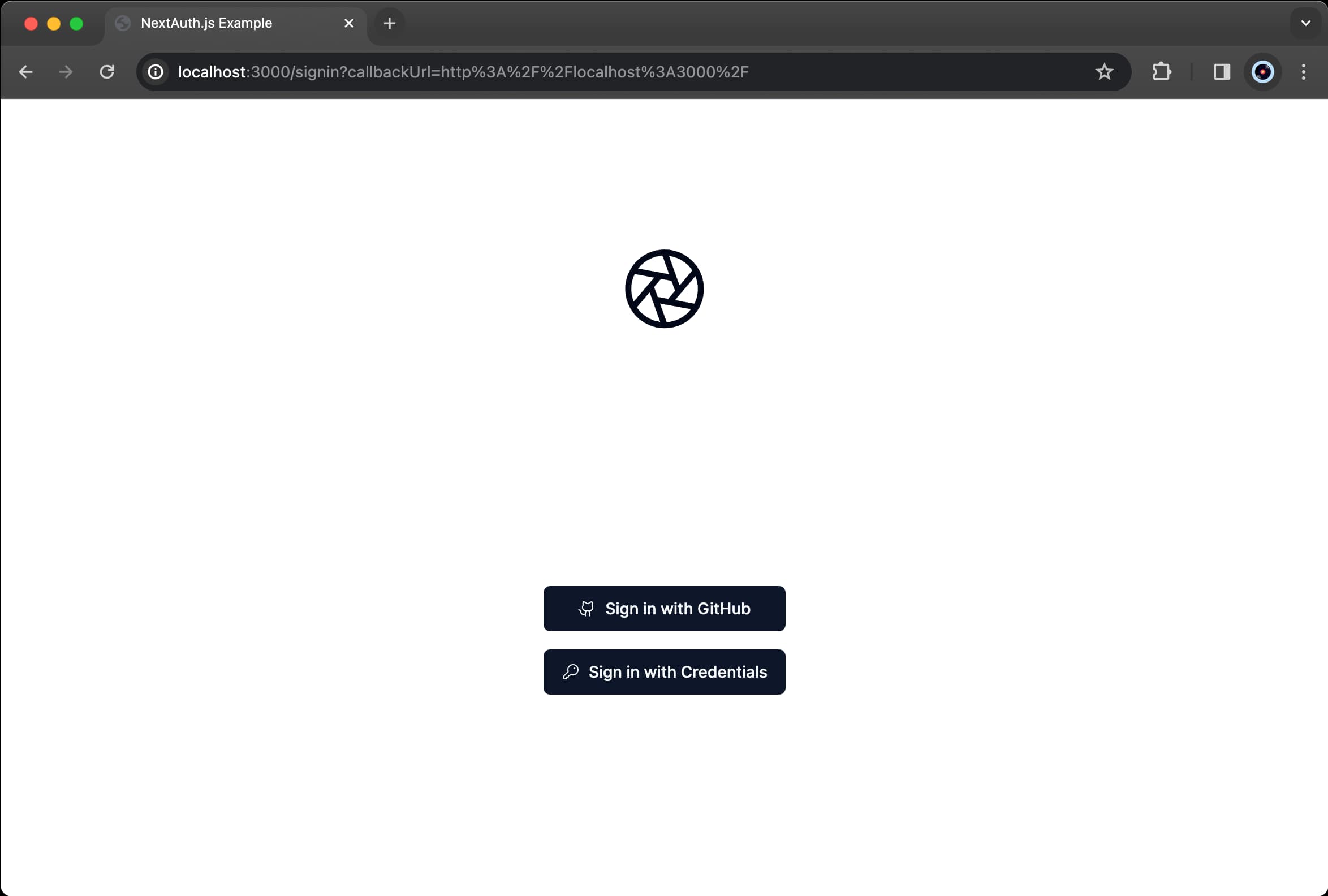